How do I setup Jumpstart Pro?
I’ve launched every one of my Ruby on Rails projects using Jumpstart Pro since mid 2020; both consulting client projects and personal projects. In this article I walk through how I get started each time.
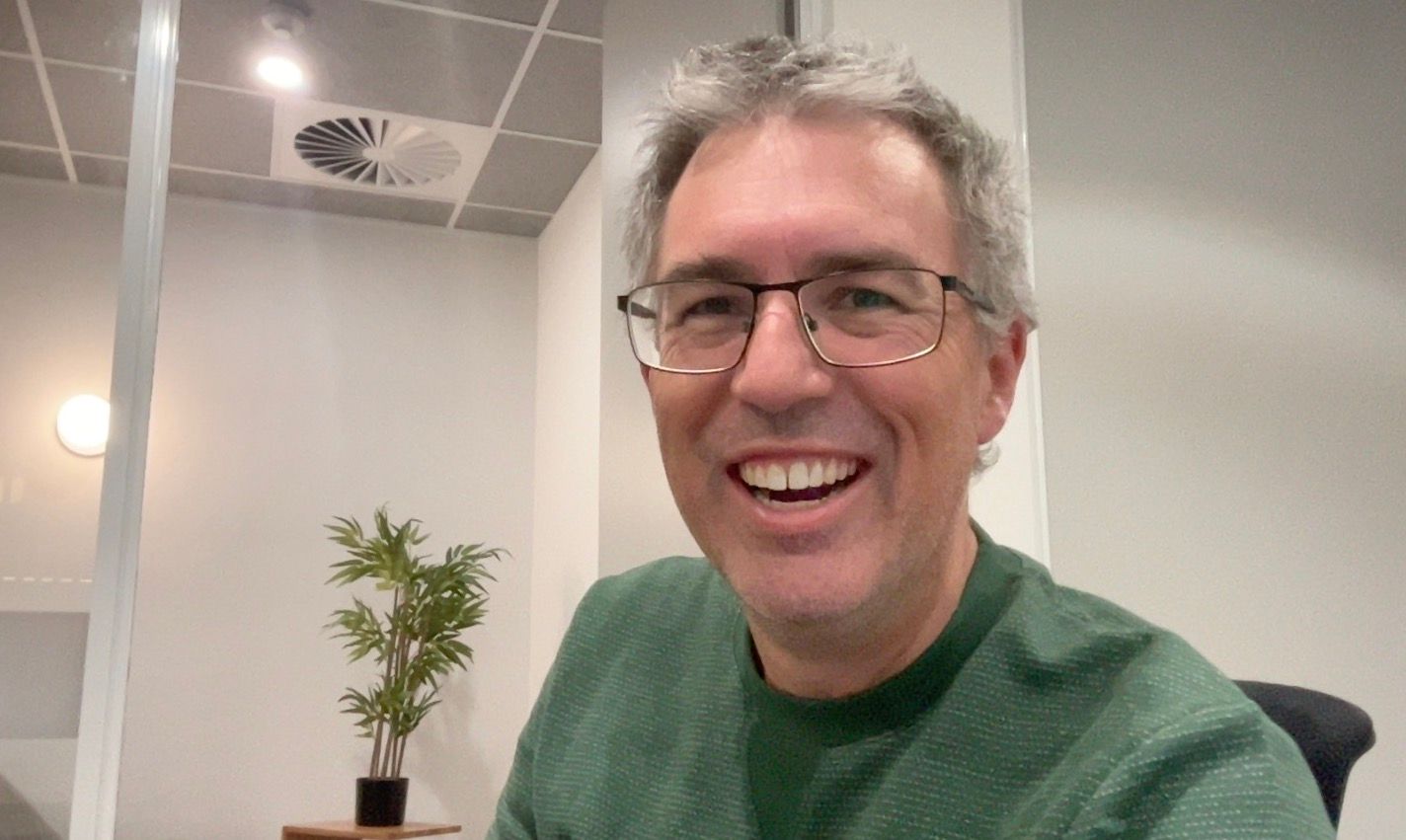
I’ve been using GoRails’ Jumpstart Pro to launch every one of my Ruby on Rails since mid 2020. I follow along with daily or weekly updates to JSP, and have made dozens of improvements to JSP. And yet, each time I start a new Rails project, using JSP, I have to remind myself: how do I setup Jumpstart Pro?
In this article, I document the steps I repeat each time I get started, including changes I copy across from my past projects, and things I hack out.
In referencing Jumpstart Pro I am only discussing the Ruby on Rails variant.
Buying a license
The delightful Jumpstart Pro magic is hidden behind a paid license.
- Visit https://jumpstartrails.com/ and click Get Started.
- Create an account. By the way, this experience of creating an account is part of Jumpstart Pro. Your apps’ account creation page will look very similar.
- Pick a license - Single Site or Unlimited - and pay for it. Any number of developers can work on your JSP-based apps; licensing is based on 1 app, or unlimited apps.
- Link your account to Github.
- You can now
git clone
the repository. You can also raise Issues, and create Pull Requests, on the repository.
Getting started
I have a clone of this repo on my laptop. I use this to watch for new changes each day or week. It’s where I also propose upstream pull requests to JSP.
mkdir -p ~/workspace
cd ~/workspace
git clone https://github.com/jumpstart-pro/jumpstart-pro-rails
When I want to create a new application I will clone from this local repo.
Let’s assume our app is called books
.
cd ~/workspace
git clone ~/workspace/jumpstart-pro-rails books
cd books
bin/setup
The bin/setup
script will attempt to install dependencies using homebrew, which I believe works on both MacOS and Linux. Primarily we want:
- PostgreSQL
- Redis
Personally I use Postgres.app for MacOS to manage my local postgresql servers, rather than homebrew. I don’t recall why.
When bin/setup
is finished, it will have:
- Downloaded many many RubyGems
- Downloaded many many npm packages
- Created databases for development and testing
The default name for the databases is jumpstart_development
and jumpstart_test
which is hard-coded in config/database.yml
Except, before I run bin/setup
I always change config/database.yml
to have unique database names so it doesn’t clash with my other JSP apps. I've proposed this in PR #684.
Here’s a striped down version without comments:
# config/database.yml
<% app_name = File.basename(Rails.root).split('-').first %>
default: &default
adapter: postgresql
encoding: unicode
pool: <%= ENV.fetch("RAILS_MAX_THREADS") { 5 } %>
development:
<<: *default
database: <%= app_name %>_development
test:
<<: *default
database: <%= app_name %>_test
production:
<<: *default
database: <%= app_name %>_production
Run bin/setup
again to create the databases, and migrate them.
Running the tests
Your new JSP app comes with a lot of tests for all the code you don’t have to write. There are two commands to run for the fast tests and the slow, system tests:
rails test
rails test:system
It’s nice to see the tests run and pass.
Any tests that fail from now onwards is your fault. That’s nice to know.
Running the app
To run the app:
bin/dev
This will run 3+ different processes that are described in Procfile.dev
:
web: bin/rails server -p $PORT
css: yarn build:css --watch
js: yarn build --reload
Any changes to your CSS or JavaScript will be automatically recompiled and your local dev app will reload them.
When we configure background workers with sidekiq, or turn on payments with Stripe, this Procfile.dev
file will be updated to also run sidekiq
and stripe
applications.
The application is now running on port 3000 at http://localhost:3000
Configuring
One of the nice features of Jumpstart Pro is the built-in configuration UI for you as a developer.
When you visit http://localhost:3000 for the first time, you’re taken straight to this config UI:
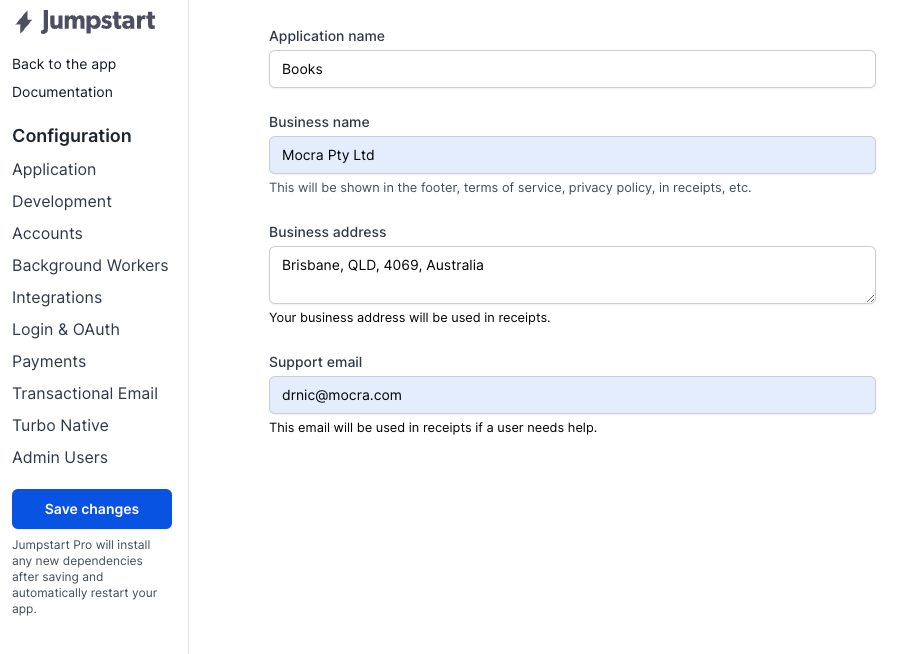
You can enter this information now, or later. To proceed press “Save changes” button.
That’s right. Whenever you update your configuration, your app — or the JSP part of your app — will update some files, perform maintenance, and restart your local app.
Subsequently, we can get back to the Configuration section by pressing “Configure Jumpstart” or visiting http://localhost:3000/jumpstart
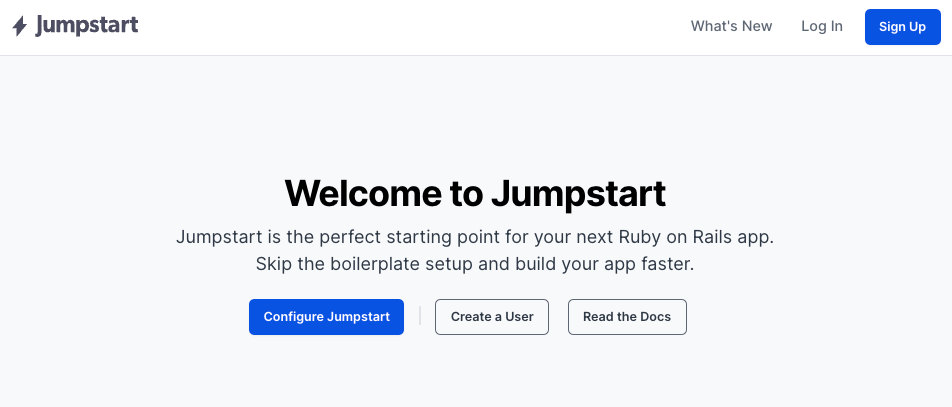
I always start my JSP apps with one other default configuration: background jobs.
Background jobs with Sidekiq
You’ll have background jobs in your app. Either implicitly: sending email, or pushing turbo broadcasts. Or explicitly: creating new jobs for your app’s code.
I use sidekiq. I see there are other options. I haven’t tried them.
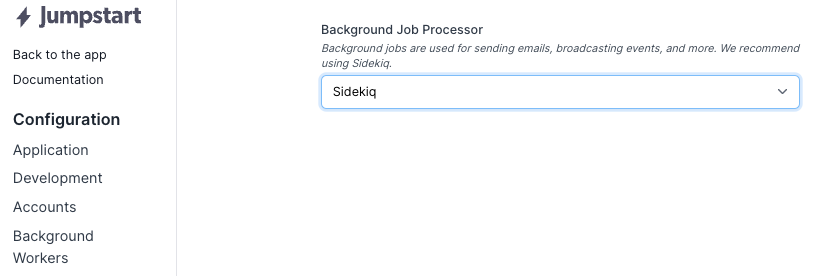
After “Save changes”, my Procfile.dev
will be updated to include worker
process:
web: bin/rails server -p $PORT
css: yarn build:css --watch
js: yarn build --reload
worker: bundle exec sidekiq
However, the app restart does not start the new worker process.
So, I need to stop the bin/dev
command and restart it.
bin/dev
At the time of writing, I also need to modify config/sidekiq.yml
to change the :queues:
configuration to weighted priorities. Learn more in sidekiq repo config.rb
. I’ve submitted a PR for this change.
:queues:
- ['critical', 10]
- ['noticed_web', 6]
- ['mailers', 5]
- ['active_storage_analysis', 4]
- ['default', 2]
- ['action_mailbox_routing', 2]
- ['active_storage_purge', 1]
- ['action_mailbox_incineration', 1]
Future configuration
Later on I’ll pick and configure:
- ActiveStorage blog storage, such as AWS S3;
- A payments system, such as Stripe;
- An outbound email system such as Mailgun;
- A bug tracking system such as Honeybadger;
- App monitoring such as Skylight; etc.
Admin user
Whilst JSP Configuration UI provides an “Admin Users” section to provision an initial User with admin: true
privileges, I prefer to put this into my db/seeds.rb
file to it is recreated again and again when I want to flush my dev database.
admin = User.find_or_create_by(email: "drnic@mocra.com") do |user|
user.name = "Dr Nic Williams"
user.password = "secret1!"
user.password_confirmation = "secret1!"
user.terms_of_service = true
user.admin = true
end
Jumpstart.grant_system_admin!(admin)
I can now create my admin user:
rails db:seed
Back in the app, I can now login.
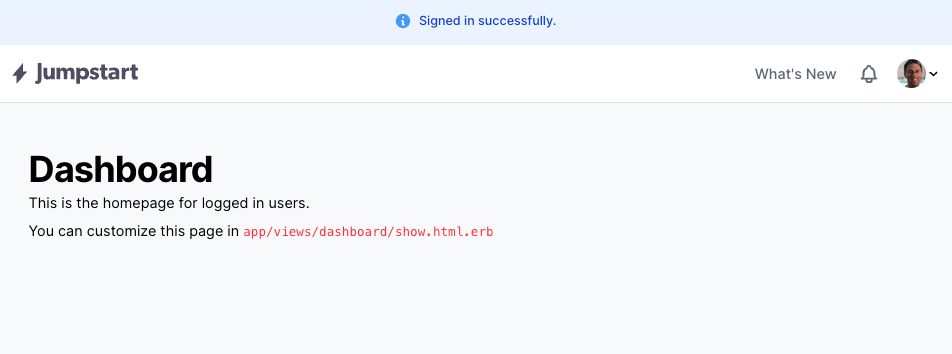
Code styles and continual linting
I want all my code, across all my files, across all my projects, to look similar. That is, I want a code style, and I care more about having a code style enforced than the specifics of the code style. So I let someone else determine my code style. Specifically I entrust this to Justin Searls, via his tool Standard Ruby, aka standardrb
, aka standard
.
For my HTML ERb files I use erblint
.
The good news is that Jumpstart Pro already has standard
and erblint
gems included in the Gemfile
and efforts are made to ensure all files, and generated files, are already validate.
To confirm that all ruby files in our new application already pass code style tests:
standardrb
If it exits, and says nothing, then that’s great news.
Next, we want to ensure standardrb
command is run before any future git commits.
JSP includes another helpful tool overcommit
that sets up git hooks. Look in the .overcommit.yml
file to see what you’ll get for free:
- It runs
standardrb --fix
which will fail if any files need fixing; but will try to fix easy changes. - It runs
erblint
to try to run code style checks on.html.erb
files.
To install our overcommit git hooks, run the following command once:
overcommit --install
NOTE: Git hooks are local only, so each new developer on the project must run overcommit
locally. If you discover that commits are landing in the project that are failing standardrb
or erblint
lint checks, it is because someone hasn’t run overcommit --install
yet.
As a bonus, I’ve also started using some standardrb
plugins for minitest and rails. See two PRs for more details #681 and #682.
Minitest v RSpec
I used to like rspec. But now I like fast tests. So now I use minitest.
Your initial app, cloned from Jumpstart Pro, comes with 200+ minitest tests. If you decide to setup rspec, you might think you should rip out those tests. Don’t. Keep them. Run those tests, plus your rspec tests, in your CI pipeline.
Also, look on the internet for explanations for why you might like to stick with minitest for your own test suite.
Jumpstart Pro scaffolding generators
One of the lovely things about JSP is that rails generate scaffold
generator makes a very lovely starting place for new features.
But I do like to change the scaffolding immediately, and continue to evolve it over time.
Firstly, I configure rails to stop it generating so many files. Inside config/application.rb
I setup config.generators
:
config.generators do |g|
g.orm :active_record, primary_key_type: :bigint
# Dont generate app/assets/controller_name.scss with generators
g.stylesheets false
g.helper false
g.jbuilder false
end
Let’s create a scaffold for some Books:
rails g scaffold books name summary:text published_at:datetime account:references
After running migrations, and refreshing our app, we see the lovely JSP themed forms and UI that we get for our scaffold:
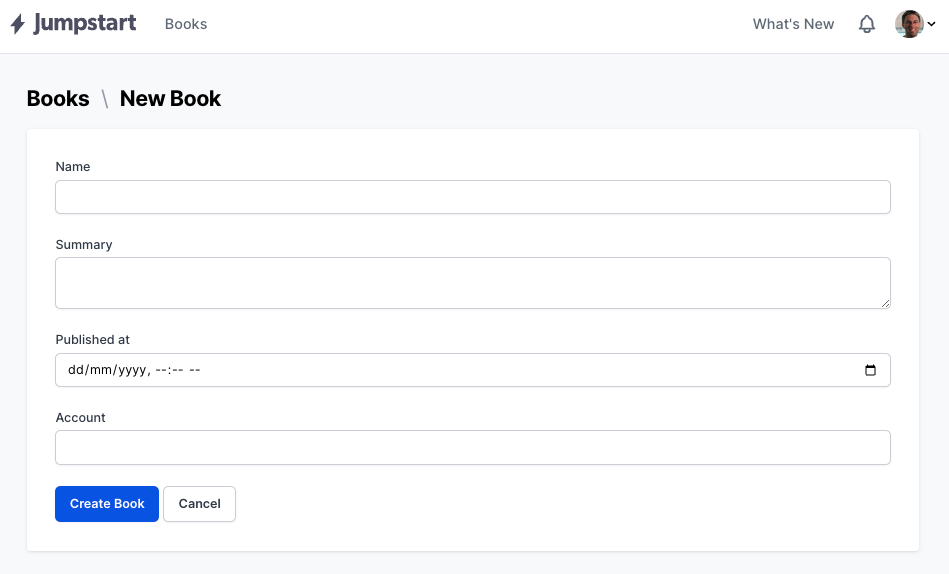
Looking inside the generated model file app/models/book.rb
we see some good things and something that I typically rip out.
# == Schema Information
#
# Table name: books
#
# id :bigint not null, primary key
# name :string
# published_at :datetime
# summary :text
# created_at :datetime not null
# updated_at :datetime not null
#
class Book < ApplicationRecord
# Broadcast changes in realtime with Hotwire
after_create_commit -> { broadcast_prepend_later_to :books, partial: "books/index", locals: {book: self} }
after_update_commit -> { broadcast_replace_later_to self }
after_destroy_commit -> { broadcast_remove_to :books, target: dom_id(self, :index) }
end
Firstly, I love the annotation at the top of the file. JSP includes the annotate
gem which will continually update the schema of the database table at the top of the model, test, and fixture files.
But I’m not a fan of the default Hotwire demo code.
If you’re new to Hotwire Turbo, then perhaps take the time to learn how it works, what this code is doing, and why it’s probably not appropriate for any application.
In short, when a new Book
is created, it will render a new chunk of HTML from app/views/books/_book.html.erb
partial, and broadcast it to … everyone who is on /books
. That’s a cool demo.
But shortly, you’ll change your code so that each Book belongs to an Account, and you only want those account users to see the new book appear. But this demo code will send that new Book partial to all users. So, I personally rip out these after_xyz_commit
commands; and I will explicitly write the Hotwire Turbo broadcast commands that I need for my app once I know what I want it to do.
Next, I remove them from the scaffold generator template lib/templates/active_record/model/model.rb.tt
so that they don’t appear again.
Have a look around the lib/templates
and lib/generators
folders. There is some lovely work in here, and its partly what you paid for when you bought JSP.
And you can edit the generators so that when you create new models or scaffolds etc, then they will automatically do what you want them to do. As you figure out the UI theme for your app, consider coming back to the lib/templates/erb/scaffold/*.html.erb.tt
files, and making the same changes into the scaffold generator templates.
Living with future Jumpstart Pro changes
Since JSP is distributed as a git repository, and we cloned it, then we might think about rebasing or merging upstream JSP repo into our own repo.
Personally, I don’t use git to merge upstream JSP.
After the initial git clone
I no longer use git
to bring in updates from the JSP project that I’ve paid for. That sounds odd.
I definitely still want new features and bug fixes from JSP in my apps. But I just haven’t found that an application of any complexity to be easily mergable with the original JSP.
I’ll have mangled all the .html.erb
templates, at the very least to change the Tailwind CSS classes to my own theme. I’ll add all my own app’s bespoke code into the shared Ruby classes like User, Account, and ApplicationController. I’ll change something about the login/account creation flow.
Instead, I treat new bug fixes or new whole features in JSP as new bug fixes or new whole features for my app. Do I want that bug fix? Do I want that new feature? Urgently today? Maybe one day?
Each day, or a few days a week, I change into the jumpstart-pro-rails
folder that we originally cloned above, I’ll pull down the latest commits, and I’ll have a look wants new.
cd ~/workspace/jumpstart-pro-rails
git pull
git log
Then I’ll look at each new commit. I’ll look at the recently merged Pull Requests.
Some tiny fixes I might copy or recreate into my current app immediately. Others I’ll create a ticket/TODO item for the project. And others still, I’ll just think “that’s cool, perhaps I’ll use that for another project one day”.
Will I continue to create new apps with Jumpstart Pro?
My consulting client projects and my own personal projects have benefitted hugely from being written upon Jumpstart Pro, and from the continue drip feeding of new bug fixes and new features that regularly appear.
I’ve also found that my own style of code writing, and that of JSP, have aligned. It is a very pleasant and productive partnership. I think of Chris Oliver as a part-time CTO for each and everyone of the applications that I have created or worked on. For under $1000 a year. Bargain.
Yes, the benefits from starting new projects on JSP, and continuing to upgrade my existing applications to align with JSP, will mean I keep using Jumpstart Pro. Thanks to Chris, and his growing GoRails team, for curating and expanding it.
Can Mocra and Dr Nic help me?
If you’ve started a new JSP app, or if you’d like to migrate your existing app idea across to JSP, then yes please let me know and I can try to help you be successful.
At Mocra, we’ve solved so many problems, across so many business domains, over the last 15 years, that it is very likely we can be impactful immediately in your business.
Or at the very least, it would be great to meet you and swap love stories about Jumpstart Pro and Ruby on Rails.